1. 工厂模式基本介绍
go的结构体没有构造函数,通常可以使用工厂模式来解决这个问题。
一个结构体的声明是这样的:
1 2 3 4
| package mode1 type Student struct{ Name string.. }
|
这里的Student
的首字母S
是大写的,如果我们想在其他包创建Student
的实例(比如main包),引入mode1
包后,就可以直接创建Student
结构体变量(实例)。但是问题来了,如果首字母是小写的,比如type student struct {...}
就不行了,怎么办—->工厂模式来解决
2. 使用工厂模式实现跨包创建结构体实例(变量)案例
2.1 创建目录
在项目目录下创建factory
目录,factory
目录下创建main
和model
文件夹
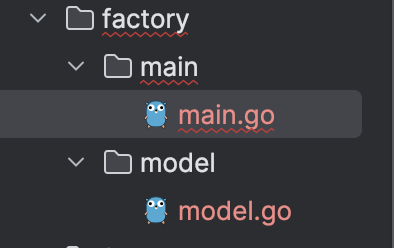
2.2 创建go.mod
2.3 编写main.go和model.go
如果model
包的结构体变量首字母大写,引入后,直接使用,没有问题
如果model
包的结构体首字母小写,引入后,不能直接使用,可以使用工厂模式解决
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| package main
import ( "factory/model" "fmt" )
func main() { stu := model.Student{ Name: "tom", Score: 66, } fmt.Println(stu) }
package model
type Student struct { Name string Score float64 }
|
2.4 使用工厂模式(Student改成student)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| package model
type student struct { Name string Score float64 }
func NewStudent(n string, s float64) *student { return &student{ Name: n, Score: s, } }
package main
import ( "factory/model" "fmt" )
func main() { stu := model.NewStudent("tom", 66.8) fmt.Println(stu) fmt.Println(*stu) fmt.Println("name=", stu.Name, "score=", stu.Score) }
|
2.5 将Score改成score
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41
| package model
type student struct { Name string score float64 }
func NewStudent(n string, s float64) *student { return &student{ Name: n, score: s, } }
func (s *student) GetScore() float64 { return s.score }
package main
import ( "factory/model" "fmt" )
func main() { stu := model.NewStudent("tom", 66.8) fmt.Println(stu) fmt.Println(*stu) fmt.Println("name=", stu.Name, "score=", stu.GetScore()) }
|