1. 抽象的基本介绍
在前面定义结构体的时候,实际上就是把一类事物共有的属性(字段)和行为(方法)提取出来,形成一个物理模型(结构体)。这种研究问题的方法称为抽象。
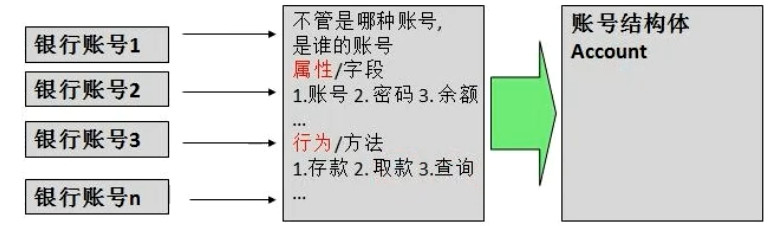
2. 实例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75
| package main
import "fmt"
type Account struct { AccountNo string Pwd string Balance float64 }
func (account *Account) Deposite(money float64, pwd string) { if pwd != account.Pwd { fmt.Println("你输入的密码不正确") return } if money <= 0 { fmt.Println("你输入的金额不正确") return } account.Balance += money fmt.Println("存款成功") }
func (account *Account) WithDraw(money float64, pwd string) { if pwd != account.Pwd { fmt.Println("你输入的密码不正确") return } if money <= 0 || money > account.Balance { fmt.Println("你输入的金额不正确") return } account.Balance -= money fmt.Println("取款成功") }
func (account *Account) Query(pwd string) { if pwd != account.Pwd { fmt.Println("你输入的密码不正确") return } fmt.Printf("你的账号=%v,余额=%v\n", account.AccountNo, account.Balance) }
func main() { account := Account{ AccountNo: "test", Pwd: "test", Balance: 100.0, } account.Query("test") account.Deposite(200.0, "test") account.WithDraw(150.0, "test") account.Query("test") }
|